Far too often, we hear developers say that interacting with Cardano’s underlying infrastructure can be quite challenging, especially if you’re unfamiliar with its native language, Haskell. To simplify development on Cardano, the community has widely invested in projects that would bridge the gap between Cardano and the commonly-used programming languages. One such project is Ogmios, which according to the 2024 Cardano Developer Survey, topped the list of the most used middleware services by Cardano developers. As a lightweight bridge interface to the Cardano’s blockchain node, Ogmios facilitates seamless interaction with Ouroboros’ mini-protocols.
In this article, we’ll explore what Ogmios is, how it works, and how developers can use it to simplify app development on Cardano.
What is Ogmios?
Ogmios is a protocol translation service that acts as an intermediary between a Cardano node and external applications. Written in Haskell, it communicates natively with the Cardano node while exposing a more accessible interface for developers that’s based on WebSockets and JSON/RPC. This design eliminates the need to write Haskell code or directly wrestle with Cardano’s Ouroboros mini-protocols, making it easier for developers to interact with the blockchain using languages they already know, such as JavaScript, Python, or C++.
Think of Ogmios as a translator: it takes the complex, Haskell-based communication of a Cardano node and converts it into a format that’s widely understood across the development community. Ogmios was first created in 2020, and since then, it has evolved alongside Cardano, supporting all major protocol updates and eras, from Shelley to Conway. The active community and consistent updates around Ogmios continue to ensure that it remains a dependable component of Cardano’s technology stack.
Ogmios provides a language-agnostic APIs which can be implemented using any WebSocket or HTTP client. There are different SDKs to meet your needs as a developer, regardless on the language of your choice. Most of these are built and supported by the community, with the latest being the .NET client built by Dave after successfully delivering the OgmiosDotnet Fund 12 project
Why Use Ogmios?
The cardano-node is the backbone of the network, handling everything from block synchronization to transaction validation. However, its interface is tailored for Haskell developers and relies on custom communication methods that can be a barrier for those working in other languages. Ogmios addresses this challenge by providing:
- Language agnostic: Ogmios eliminates the need for complex Haskell or CLI-based interactions. With the service, you can use any language that supports WebSockets or HTTP thereby broadening the pool of developers that can build on Cardano.
- Real-time data access: Ogmios facilitates direct interaction with Cardano’s networking layer and delivers live updates from the blockchain, such as new blocks or transaction states. This makes it ideal for applications requiring real-time interaction.
- Lightweight design: Ogmios doesn’t require developers to run a full node or rely on heavy APIs. It’s resource efficient in that it simplifies blockchain queries and event-driven workflows, thereby reducing the overhead for developers.
- Flexibility: It supports a wide range of use cases, from wallets and explorers to smart contract backends, by exposing the full capability of the Cardano node.
- Easier debugging: When working with smart contracts or transactions, understanding execution costs or chain state is critical. Ogmios provides detailed, real-time insights—like script evaluation or chain tip data—in a simple JSON format, making it easier to debug and optimize your code.
- Community-driven platform: As an open-source tool, Ogmios benefits from a growing ecosystem of client libraries and community support. Whether you need a quick TypeScript integration or a custom solution in another language, you’re not locked into a proprietary system—you can adapt it to your needs.
Ogmios supports key functionalities like chain synchronization, transaction submission, ledger state queries, and mempool monitoring. Its WebSocket-based communication enables real-time, bidirectional messaging, making it ideal for applications that require high-speed interactions with the Cardano network such as wallets or monitoring tools.
For developers building decentralized applications (DApps), tools like Ogmios are essential for streamlining development and accelerating innovation on Cardano. You can use any of the open-source client libraries to integrate Cardano functionalities seamlessly into your projects.
How does Ogmios Work?
Ogmios sits between a running cardano-node
instance and client applications, translating raw blockchain data into an easy-to-use JSON format. The process involves:
- WebSocket Server: Establishes a persistent connection for fast, real-time blockchain queries.
- Request Handling: Processes JSON/RPC requests, such as querying the current slot or submitting transactions.
- Ouroboros Mini-Protocols: Directly interfaces with key blockchain protocols, including:
- ChainSync: For retrieving block headers and new transactions.
- TxSubmission: For submitting signed transactions to the network.
- LocalStateQuery: For querying the ledger state.
Setting Up Ogmios
To start using Ogmios, you’ll need a running Cardano node, as Ogmios relies on it to fetch blockchain data. The simplest way to get started is with Docker, which allows you to orchestrate both a Cardano node and an Ogmios instance seamlessly. Here’s a basic setup guide:
- Install Docker and Docker Compose: Ensure these tools are installed on your system.
- Clone the Ogmios Repository: Grab the source code using
git clone --depth 1 https://github.com/CardanoSolutions/ogmios.git
and navigate to the ogmios directory. - Run with Docker Compose: Use the provided compose file to launch both services by running
docker-compose up
. This sets up a Cardano node connected to the mainnet (or another network, if configured) and an Ogmios server listening on localhost:1337. - Verify the Setup: Access
http://localhost:1337/health
to check the server’s status and metrics in real time.
Alternatively, you can build Ogmios from source using Haskell’s Cabal tool, though this requires additional dependencies like GHC (Glasgow Haskell Compiler). Pre-compiled binaries are also available for Linux systems, offering a quick-start option for those who prefer not to build locally.
Developing with Ogmios
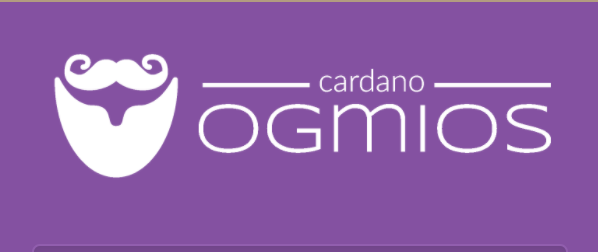
Once Ogmios is running, you can interact with it via its WebSocket API. This API supports a variety of methods, such as querying the chain tip, submitting transactions, or evaluating smart contract execution cost. All these are formatted in JSON for easy parsing. Here’s how you can start building:
Step 1. Choose a client library
Ogmios provides official client libraries in languages like TypeScript, with community-supported options for Python, Go, Kotlin, Java, and more in development (e.g., C++ and .NET). For example, the TypeScript client simplifies connecting to Ogmios and handling responses. Install it with:
npm install @cardano-ogmios/client
Step 2: Connect to the blockchain
Using the TypeScript client, you can connect to Ogmios and fetch the current chain tip:
import { createConnection } from '@cardano-ogmios/client';
async function getChainTip() {
const connection = createConnection({ host: 'localhost', port: 1337 });
const client = await connection.start();
const tip = await client.chainTip();
console.log('Current chain tip:', tip);
await client.shutdown();
}
getChainTip();
This snippet establishes a WebSocket connection, retrieves the latest block information, and closes the connection cleanly.
Step 3: Build real-time applications
Ogmios excels at real-time updates. For instance, you could monitor new blocks as they’re added:
async function watchBlocks() {
const connection = createConnection({ host: 'localhost', port: 1337 });
const client = await connection.start();
await client.nextBlock((direction, tip, point) => {
if (direction === 'forward') {
console.log('New block:', point);
}
});
}
watchBlocks();
This code listens indefinitely for new blocks, printing their details as they arrive, which is a typical foundation for explorers or live dashboards.
Step 4: Handle transactions and smart contracts
Ogmios also supports transaction submission and script evaluation, crucial for DApp development. You can submit a serialized transaction or evaluate the execution units of a smart contract without submitting it, helping you optimize costs before deployment.
Potential Use Cases for Ogmios
Ogmios unlocks a wide range of development opportunities on Cardano, from low-level blockchain interactions to high-level application logic.These include
- Blockchain indexing: Blockchain explorers and analytics platforms require real-time access to blockchain data. Ogmios allows developers to subscribe to new block headers and transactions in a highly efficient manner.
- Smart contract monitoring: Developers need to monitor on-chain smart contract executions and events. By leveraging the
LocalStateQuery
protocol, Ogmios allows efficient querying of contract state changes:
- Wallet and dApp development: Ogmios makes it easy for wallet applications and decentralized apps (dApps) to interact with Cardano’s ledger. A wallet app can query a user’s account balance efficiently:
- Transaction automation: For large-scale operations, such as staking pool distributions or token airdrops, Ogmios enables automated transaction submissions with minimal overhead. The following code shows how a batch of transactions can be submitted:
- Light clients for mobile and web apps: Mobile wallets and web-based dApps require an efficient way to fetch blockchain data without running a full node. Ogmios provides an ideal interface, allowing light clients to retrieve only necessary information without consuming excessive bandwidth or storage.
In short, Ogmios empowers developers by removing friction, speeding up workflows, and offering unparalleled access to Cardano’s features. It’s the bridge that turns a challenging platform into an approachable one, enhancing your ability to innovate. Whether you’re creating a wallet, explorer, or DApp, Ogmios is a tool worth exploring for any builder looking to accelerate their development journey on Cardano.